Top 50 C Coding Interview Questions and Answers (2024)
C is the most popular programming language developed by Dennis Ritchie at the Bell Laboratories in 1972 to develop the UNIX operating systems. It is a general-purpose and procedural programming language. It is faster than the languages like Java and Python. C is the most used language in top companies such as LinkedIn, Microsoft, Opera, Meta, and NASA because of its performance. To get into these companies and other software companies, you need to master some important C coding questions to crack their C Online Assessment round and coding interview.
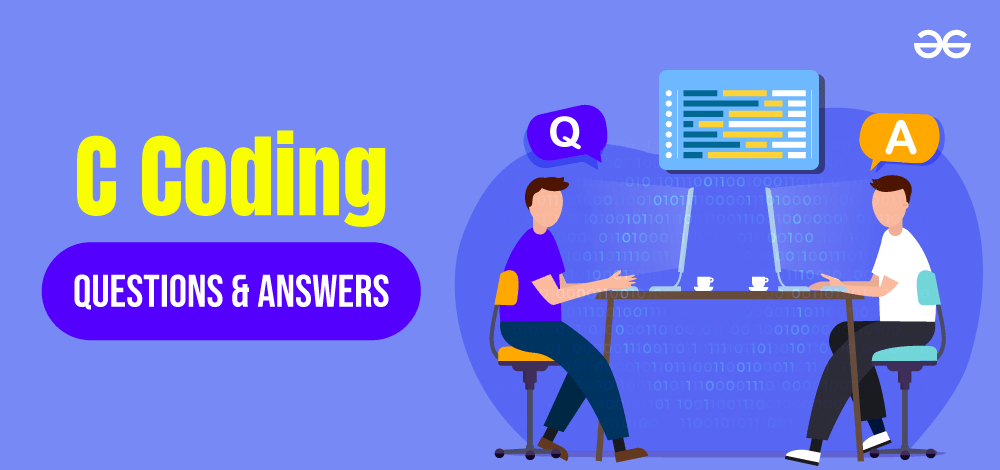
C Coding Interview Questions and Answers
This article on C Coding Interview Questions offers a comprehensive collection of practice questions suitable for both beginners and advanced learners.
List of 50 C Coding Interview Questions and Answer
Here is a list of 50 C coding interview questions and answers:
1. Find the largest number among the three numbers.
Output
2. Write a Program to check whether a number is prime or not.
Output
Is a Prime Number
3. Write a C program to calculate Compound Interest.
Output
Compound Interest is : 714.830823
4. Write a Program in C to Swap the values of two variables without using any extra variable.
Output
x: 10 , y: 20 x: 20 , y: 10
5. Write a Program to Replace all 0’s with 1’s in a Number.
Output:
112311
6. Write a Program to convert the binary number into a decimal number.
Output
7. Write a Program to check if the year is a leap year or not.
Output
2000 is a leap year. 2002 is not a leap year. 2008 is a leap year.
8. Write a program to Factorial of a Number.
Output
Factorial of 13 is 6227020800 Factorial of 9 using recursion:362880
9. Write a Program to Check if a number is an Armstrong number or not.
Output
False True
10. Write a program to Find all the roots of a quadratic equation in C.
Output:
Roots are real and different
15.937254
0.062746
11. Write a Program to reverse a number.
Output
Initial number:15942 24951 after reverse using iteration 15942 after again reverse using recursion
12. Check whether a number is a palindrome.
Output
13431 is palindrome 12345 is not a palindrome
13. Write a C Program to check if two numbers are equal without using the bitwise operator.
Output
1 is not equal to 2
14. Write a C program to find the GCD of two numbers.
Output
GCD of 98 and 56 is 14
15. Write a C program to find the LCM of two numbers.
Output
16. Write a C Program to find the Maximum and minimum of two numbers without using any loop or condition.
Output
max = 55 min = 23
17. Write a Program in C to Print all natural numbers up to N without using a semi-colon.
Output
1 2 3 4 5 6 7 8 9 10
18. Write a Program to find the area of a circle.
Output
Area is 78.550000
19. Write a Program to create a pyramid pattern using C.
Output
20. Write a program to form Pascal Triangle using numbers.
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
Output
1 1 1 1 2 1 1 3 3 1 1 4 6 4 1
21. Write a Program to return the nth row of Pascal’s triangle.
Output
1,5 ,10 ,10 ,5 ,1
22. Write a program to reverse an Array.
Output
5 4 3 2 1
23. Write a program to check the repeating elements in C.
Output
1 3 5
24. Write a Program to print the Maximum and Minimum elements in an array.
Output
Smallest: 2 and Largest: 83
25. Write a Program for the cyclic rotation of an array to k positions.
Output
3 4 5 1 2
26. Write a Program to sort First half in Ascending order and the Second in Descending order.
Output
11 16 23 83 73 59 42
27. Write a Program to print sums of all subsets in an array.
Output
6 3 4 1 5 2 3 0
28. Write a Program to Find if there is any subarray with a sum equal to 0.
Output
True subarray with 0 sum is possible
29. Write a C program to Implement Kadane’s Algorithm
Output
Maximum contiguous sum is 7 Starting index 2 Ending index 6
30. Write a Program to find the transpose of a matrix.
Output
Result matrix is 1 2 3 1 2 3 1 2 3 1 2 3
31. Write a Program to Rotate a matrix by 90 degrees in the clockwise direction in C.
Output
Orignal Matrix: 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 Matrix after rotation: 13 9 5 1 14 10 6 2 15 11 7 3 16 12 8 4
32. Write a Program to find the Spiral Traversal of a Matrix in C.
Output
1 5 9 13 14 15 16 12 8 4 3 2 6 10 11 7
33. Write a program to count the sum of numbers in a string.
Output
34. Program to calculate the length of the string.
Output
length using strlen:13 length using iteration:13 length using recursion:13
35. Write a program to check string is a palindrome.
Output
GeekeeG is a palindrome Checking GeeksforGeeks using recursive approach Not a Palindrome
36. Write a program to print all permutations of a given string in lexicographically sorted order in C.
Output
123 132 213 231 312 321
37. Write a program to calculate the Power of a Number using Recursion in C.
Output
1024
38. Write a Code to print the Fibonacci series using recursion.
Output
Fibonacci using recursion of 9:34 Fibonacci using iteration of 11:144
39. Write a Program to find the HCF of two Numbers using Recursion.
Output
GCD of 192 and 36 is 12
40. Write a Program in C to reverse a string using recursion.
Output
Orignal string:Geeks for Geeks Reverse the string(iteration):skeeG rof skeeG Using recursion for reverse:Geeks for Geeks
41. Write a C Program to search elements in an array.
Output
Element is present at index 4
42. Write a C Program to search elements in an array using Binary Search.
Output
Element is present at index 4
43. Write a C Program to sort arrays using Bubble, Selection, and Insertion Sort.
Output
Non-Sorted array: 9 4 3 11 1 5 Sorted array using Bubble sort: 1 3 4 5 9 11 Non-Sorted array: 4 3 9 1 5 11 Sorted array using Insertion Sort: 1 3 4 5 9 11 Non-Sorted array: 5 1 11 3 4 9 Sorted array using Selection Sort: 1 3 4 5 9 11
44. Write a C Program to sort arrays using Merge Sort.
Output
Given array:23 9 13 15 6 7 Sorted array :6 7 9 13 15 23
45. Write a C Program to sort arrays using Quick Sort.
Output
Unsorted Array:28 7 20 1 10 3 6 Sorted array :1 3 6 7 10 20 28
46. Write a program to sort an array using pointers.
Output
4 7 12 13 22
47. Write a C program to Store Information about Students Using Structure
Output
Student Records: Name : Geeks1 Roll Number : 1 Age : 10 Name : Geeks2 Roll Number : 2 Age : 11 Name : Geeks3 Roll Number : 3 Age : 13
48. Write a C Program To Add Two Complex Numbers Using Structures And Functions.
Output
x = 4 + 5i y = 7 + 11i sum = 11 + 16i
49. Write a C Program to add Two Distance Given as Input in Feet and Inches
Output
Feet Sum: 31 Inch Sum: 5.70
50. Write a C program to reverse a linked list iteratively
Output
Given linked list 25 19 14 10 Reversed linked list 10 14 19 25
Conclusion
In this C coding interview questions and answers, we’ve compiled a wide-range of practice questions suitable for individuals at all levels, from beginners to advanced learners. Exploring these questions and their solutions will not only enhance your proficiency in C but also prepare you for a successful coding interview experience.
C Coding Interview Questions – FAQs
Q: What are the most common C coding interview questions?
- C syntax and semantics
- Data structures and algorithms
- Memory management
- Pointers
- File I/O
- Reverse a linked list.
- Implement a binary search tree.
- Write a function to find the maximum element in an array.
- Explain the difference between a pointer and an array.
- What is the difference between a function declaration and a function definition?
- How do you allocate memory on the heap?
- How do you free memory that has been allocated on the heap?
- What is a dangling pointer?
- How do you read and write data to a file?
Q. Who can benefit from these C coding interview questions and answers?
These questions are designed to benefit anyone preparing for a C coding interview. Whether you’re a beginner looking to learn the fundamentals or an experienced programmer aiming to enhance your C skills, this resource can assist you in your preparation.
Q: How can I use these questions effectively in my interview preparation?
Start by assessing your current level of expertise in C programming language. Then, you can use these questions to gradually build your skills up and knowledge. Practice solving them on your own, and review the explanations to ensure a thorough understanding.